I have two triggers, get_entity and get_project, that I’d like relate in a parent-child hierarchy. I’ve tried to adapt X for my needs.
I created the get_entity trigger:
const perform = async (z, bundle) => {
return t
{ id: 1, name: 'Entity 1' },
{ id: 2, name: 'Entity 2' }
];
};
module.exports = {
operation: {
perform: perform,
outputFields: l
{ key: 'id', type: 'integer' },
{ key: 'name', type: 'string' },
],
},
display: {
description: 'Get a list of entities.',
hidden: true,
label: 'Get Entities',
},
key: 'get_entity',
noun: 'Entity',
};
I created the get_project trigger:
const perform = async (z, bundle) => {
const e1 = e
{ id: 1, name: 'E1 - P1' },
{ id: 2, name: 'E1 - P2' }
];
const e2 = e
{ id: 1, name: 'E2 - P1' },
{ id: 2, name: 'E2 - P2' }
];
return bundle.inputData.entity_id == 1 ? e1 : e2;
};
module.exports = {
operation: {
perform: perform,
inputFields: l
{
key: 'entity_id',
label: 'Entity ID',
type: 'integer',
required: true,
dynamic: 'get_entity.id.name',
},
],
outputFields: l
{ key: 'id', type: 'integer' },
{ key: 'name', type: 'string' },
],
},
display: {
description: 'Get a list of projects.',
hidden: true,
label: 'Get projects',
},
key: 'get_project',
noun: 'Project',
};
I created the lorem_ipsum action:
const perform = async (z, bundle) => {
return {id: 1, name: 'Lorem Ipsum'};
};
module.exports = {
display: {
description: 'lorem ipsum',
hidden: false,
label: 'Lorem Ipsum',
},
key: 'lorem_ipsum',
noun: 'Lorem',
operation: {
inputFields: l
{
key: 'project_id',
label: 'project',
type: 'integer',
dynamic: 'get_project.id.name',
required: true,
list: false,
altersDynamicFields: false,
},
],
outputFields: l
{ key: 'id' },
{ key: 'name' },
],
perform: perform,
},
};
I created a Zap and added my Lorem Ipsum action. When I try to select a project, I get this error:
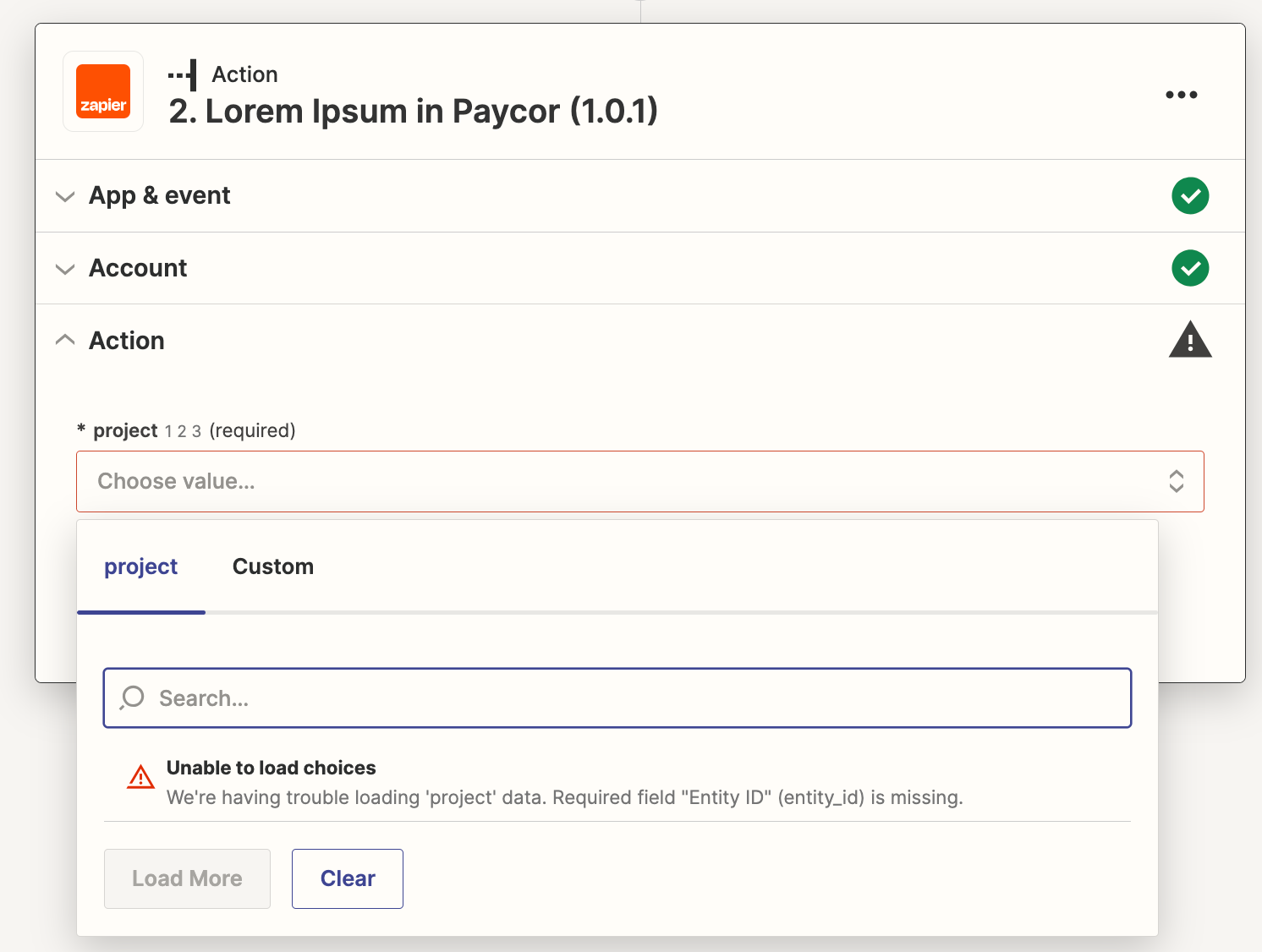
What am I not understanding?