Hey guys, first post here and hopefully someone can help me.
I have an input field which I am using to make a request to and get a response that looks like so:
{
"StatusId": 1,
"StatusDescription": "Success",
"DetailedDescription": "",
"FunctionName": "api/v2/WhatsApp/GetTemplate",
"RequestId": ",wWYuJXZ05WauUncvZmbp5CMxkGchNWPl1WYOR3cvhkJyMTQzUSM1E0MlETMrYjMtIDMtQjMwITPw1WY0NVZtlGVmEzNyAzNwETPklkclNXV",
"Data": {
"TemplateId": 150351,
"TemplateName": "תבנית שיווקית בסיסית משופעת כפתורים (5)",
"MessageText": "שלום [#1#],\r\nתודה על קנייתך. \r\nאנו בטוחים שתהנה מ[#2#] החדש שלך.\r\nלהלן מידע חיוני לשימוש במוצר.\r\nשמחנו לסייע. ואנו זמינים לכל שאלה.\r\n\r\nבברכה,\r\nKirBi\r\n\r\nלהסרה השב הסר",
"TemplateParameters": [
"[#1#]",
"[#2#]"
],
"ApprovalStatus": 5,
"ApprovalStatusDescription": "New",
"Category": "UTILITY"
}
}
What I want to do is iterate over Data.TemplateParameters and create inputs accordingly..
I thought I was doing this correctly using the code editor and ended up with this code:
const options = {
url: 'https://capi.inforu.co.il/api/v2/WhatsApp/GetTemplate',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: {
"Data": {
'TemplateId': bundle.inputData.TemplateId
}
}
};
return z.request(options)
.then((response) => {
response.throwForStatus();
const results = response.content;
var res = JSON.parse(results);
var data = [
{
"key": "TemplateParameters",
"children": []
}
];
res.Data.TemplateParameters.forEach(param => {
data[0].children.push(
{
key: param,
children: [
{
key: "Type",
label: "Type",
choices: ['Text','Contact','Custom'],
type: 'string'
},
{
key: "Value",
label: "Value",
type: 'string'
}
]
}
);
});
return data;
});
The result of my push is:
[
{
"key": "TemplateParameters",
"children": [
{
"key": "[#1#]",
"children": [
{
"key": "Type",
"label": "Type",
"choices": [
"Text",
"Contact",
"Custom"
],
"type": "string"
},
{
"key": "Value",
"label": "Value",
"type": "string"
}
]
},
{
"key": "[#2#]",
"children": [
{
"key": "Type",
"label": "Type",
"choices": [
"Text",
"Contact",
"Custom"
],
"type": "string"
},
{
"key": "Value",
"label": "Value",
"type": "string"
}
]
}
]
}
]
I suspect this scheme is wrong because my end result is:
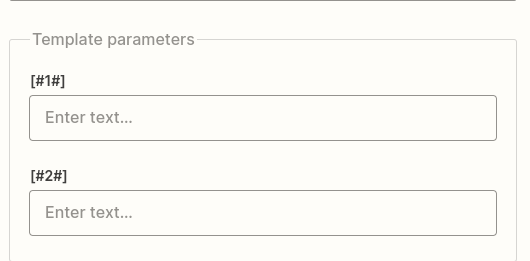
Can you spot what im doing wrong ?
Any help would be appreciated!