Im still new to JS and web-dev in general, but I am trying to use Code by Zapier blocks to run a companies API to retrieve CSV data. The API POST’s an authentication request which returns an ID string as a JSON. You then use that preauthenticated ID, appended to their base URL to GET the CSV file once it is generated. I have checked with chatGPT, and JSHint.com and am not finding any errors. Furthermore, I took screenshots of network request activity after clicking test, and according to the companies intergrations team the traffic is consistent with a successful call to the server, and response from it. This leads me to believe I am messing up something with my implementation with respect to Zapiers environment specifically, but I cant identify where from Zapiers documentation for what I imagine is lack of experience. Any help or guidance would be greatly appreciated as I feel like I am maddeningly close to the solution but cant quite get there on my own.
The code is as follows: *note: code is currently paired down to only get the ID returned for troubleshooting purposes*
output = {id: "no data"};
method: 'POST',
},
callback(error);
}
}
getID();
network request traffic:
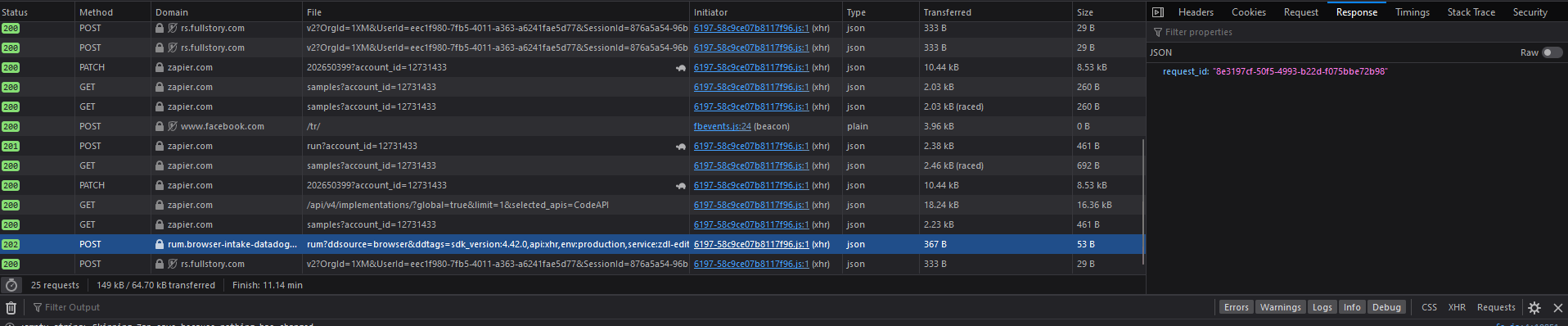
Best answer by raydeck
View original