Hello together,
we are currently facing the problem that API access with new access token fails after token was refreshed the second time.
For better testing we use very short timespan for the access token in Keycloak:
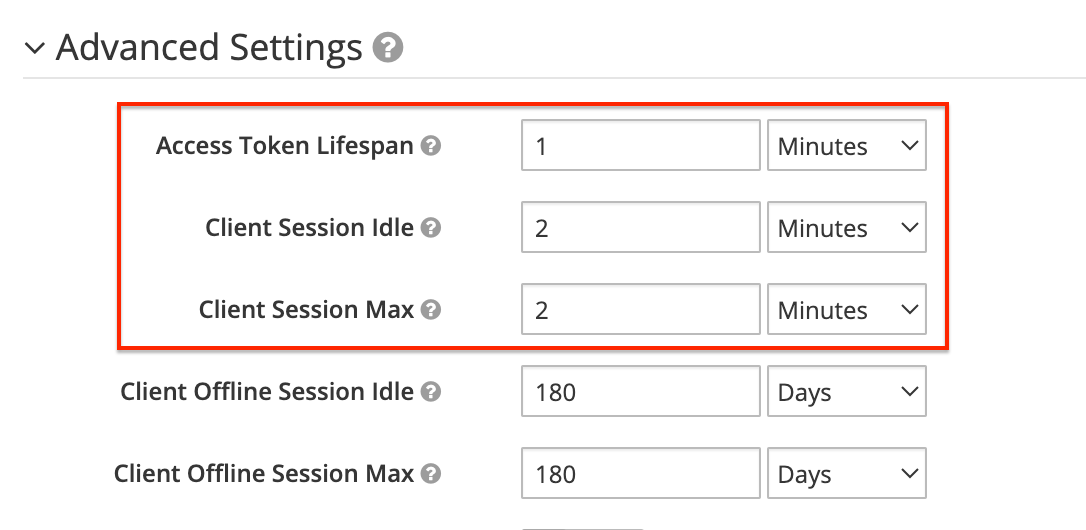
The initial OAuth2 Flow /auth endpoint is triggered succcessfully and the first token retrieved also is accepted by our API.
Then after 1 Minute (when the token expired) we get a 403 from API and trigger a RefreshAccessToken call with scope “offline_access”.
This successfully returns a new token which is accepted by our API.
Again, another minute later, our API returns 403 and RefreshAccessToken call is triggered.
This time our API refuses the Token with 403 and Zapier Zap failed with “Please reconnect your account”.
After debugging the Token from our API we noticed that this was an old token which expired already. It seems Zapier did not use the new access_token but reused the old one.
Maybe I did somethring wrong in the code on how to return the new token (we use zapier-platform-core 15.19.0):
// 'use strict';
const getAccessToken = async (z, bundle) => {
z.console.log('TEST17Dec In GetAccessToken ...');
const response = await z.request({
url: `${bundle.inputData.omnurl}/auth/realms/OMN/protocol/openid-connect/token`,
method: 'POST',
body: {
client_id: 'omn-ui',
grant_type: 'authorization_code',
scope: 'openid offline_access',
redirect_uri: bundle.inputData.redirect_uri,
code: bundle.inputData.code,
},
headers: { 'content-type': 'application/x-www-form-urlencoded' },
});
z.console.log('TEST17Dec GetAccessToken - response', response.data);
return {
access_token: response.data.access_token,
refresh_token: response.data.refresh_token,
omnurl: bundle.inputData.omnurl
};
};
const refreshAccessToken = async (z, bundle) => {
z.console.log('TEST17Dec GetRefreshToken ...');
const response = await z.request({
url: `${bundle.authData.omnurl}/auth/realms/OMN/protocol/openid-connect/token`,
method: 'POST',
body: {
client_id: 'omn-ui',
grant_type: 'refresh_token',
scope: 'openid offline_access',
refresh_token: bundle.authData.refresh_token,
redirect_uri: bundle.inputData.redirect_uri
},
headers: { 'content-type': 'application/x-www-form-urlencoded' },
});
z.console.log('TEST17Dec GetRefreshToken - response', response.data);
return {
access_token: response.data.access_token,
refresh_token: response.data.refresh_token,
omnurl: bundle.inputData.omnurl
};
};
const includeBearerToken = (request, z, bundle) => {
if (bundle.authData.access_token) {
z.console.log('TEST17Dec Added JWT Token', bundle.authData);
request.headers.Authorization = `Bearer ${bundle.authData.access_token}`;
}
return request;
};
// HTTP after middleware that checks for errors in the response.
const handleErrors = (response, z) => {
if (response.status === 403) {
z.console.log(`Middleware - Response 403: Triggering auto refresh`);
throw new z.errors.RefreshAuthError();
}
response.throwForStatus();
return response;
};
// You want to make a request to an endpoint that is either specifically designed
// to test auth, or one that every user will have access to. eg: `/me`.
// By returning the entire request object, you have access to the request and
// response data for testing purposes. Your connection label can access any data
// from the returned response using the `json.` prefix. eg: `{{json.username}}`.
const test = (z, bundle) =>
z.request({ url: `${bundle.authData.omnurl}/api/v1/search/omn-search-version` });
module.exports = {
config: {
type: 'oauth2',
oauth2Config: {
authorizeUrl: {
url: `{{bundle.inputData.omnurl}}/auth/realms/OMN/protocol/openid-connect/auth`,
params: {
client_id: 'omn-ui',
redirect_uri: '{{bundle.inputData.redirect_uri}}',
response_type: 'code',
scope: 'openid offline_access'
},
},
getAccessToken,
refreshAccessToken,
autoRefresh: true,
},
fields: [
{
helpText: 'OMN Url',
computed: false,
key: 'omnurl',
required: true,
label: 'OMN Url',
type: 'string',
},
{
key: 'access_token',
type: 'string',
computed: true,
required: false,
},
{
key: 'refresh_token',
type: 'string',
computed: true,
required: false,
},
],
test,
connectionLabel: '{{omnurl}}',
},
befores: [includeBearerToken],
afters: [handleErrors]
};