Hello,
I want to create a Zapier integration of my REST API. The endpoint of the API accepts an image as input. For testing I am using google drive to provide the image like this:
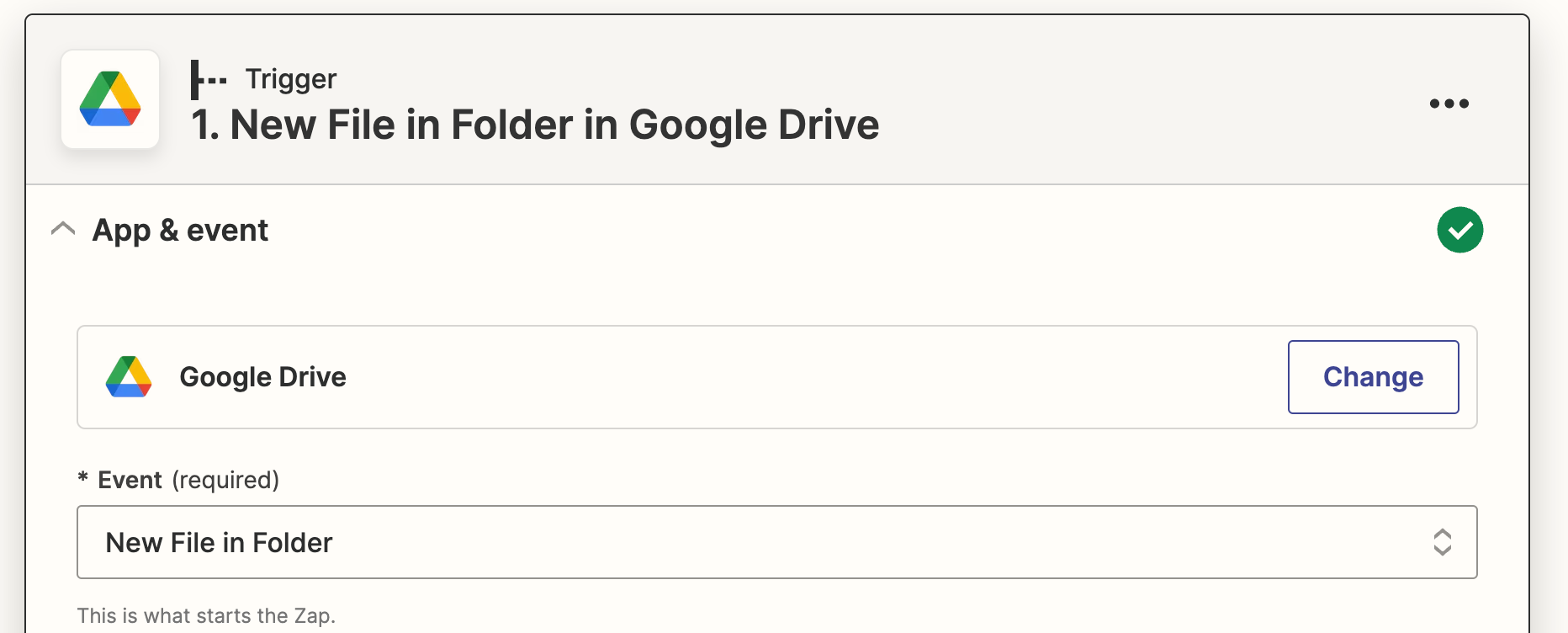
In the configuration of my integration I have the following:

The code for my integration looks like this:
const http = require('https');
const fetch = require('node-fetch');
const FormData = require('form-data');
const makeDownloadStream = (url) =>
new Promise((resolve, reject) => {
http
.request(url, (res) => {
// We can risk missing the first n bytes if we don't pause!
res.pause();
resolve(res);
})
.on('error', reject)
.end();
});
const perform = async (z, bundle) => {
const stream = await makeDownloadStream(bundle.inputData.file, z);
const formData = new FormData();
formData.append("file", stream, {
filename: "file.png" // for testing I have set the file name static
//filename: bundle.inputData.name
})
stream.resume();
const options = {
url: 'https://MY_DOMAIN_WITH_ENDPOINT',
method: 'POST',
headers: {
'Content-Type': 'application/json',
Accept: 'application/json',
'api-key': '{{bundle.authData.api-key}}'
},
params: {},
body: formData
};
return z.request(options).then((response) => {
response.throwForStatus();
const results = response.json;
// You can do any parsing you need for results here before returning them
return results;
});
};
My REST API is expecting a multipart file. Unfortunately it is complaining that the sent data is not a multipart file.
For Postman I can use the following configuration:
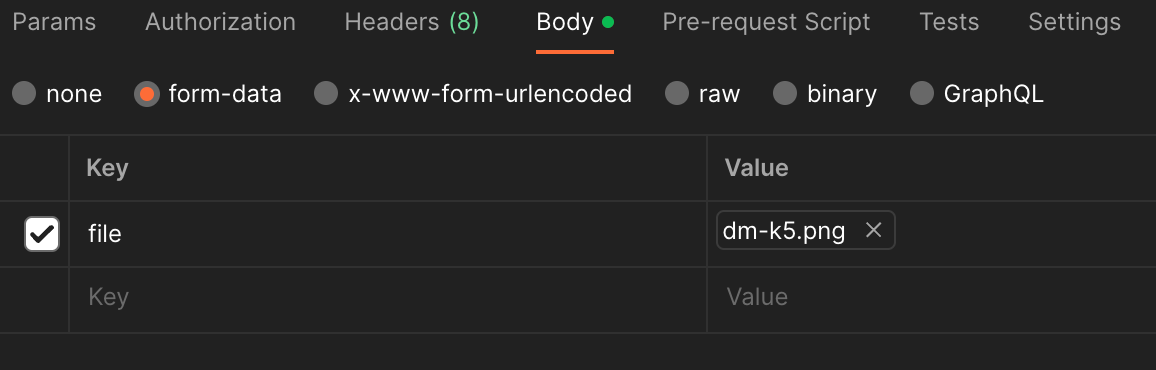
How can I modify my code to send the correct data to my REST API?
Thank you for your support!