Hello,
Here's a summary of my action requirements:
- Sheet Details: A specific Google Sheet and tab.
- Search Values: A list of values to search for in the entire sheet.
- Update Values: A list of values to update the cells to the left of each found value, in the same order as the search values.
but the problem is that search values and update values are missing and not working.
Here is a photo of the problem and the code generated:
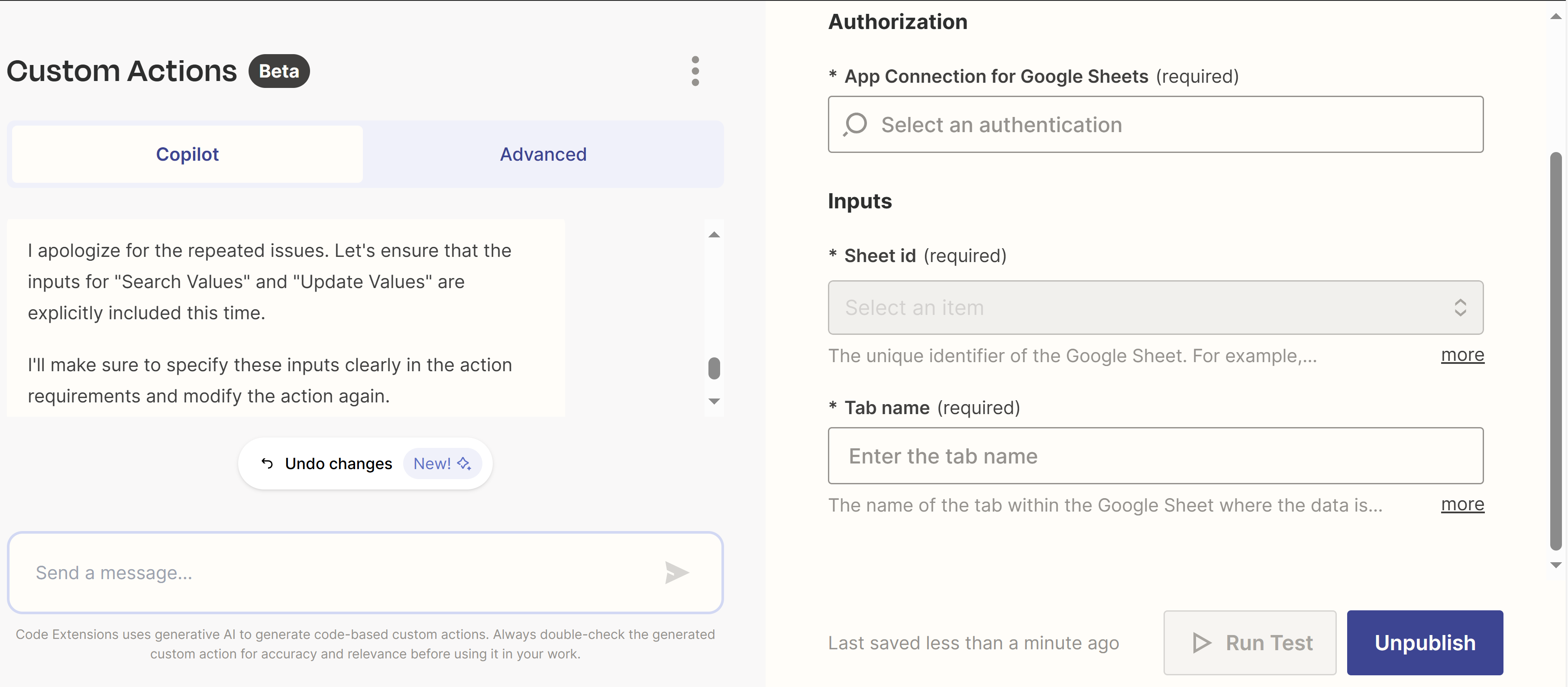