I created a Zap that pulls user data when a new membership is created in a gym app call PushPress. When I get this I want to take input data and write it to a Python script then execute the script to crate an account in another app.
Everything works great except im not a Python developer and need to write the input data to the script then execute it. the part thats not working is the ability to write that info into the script.
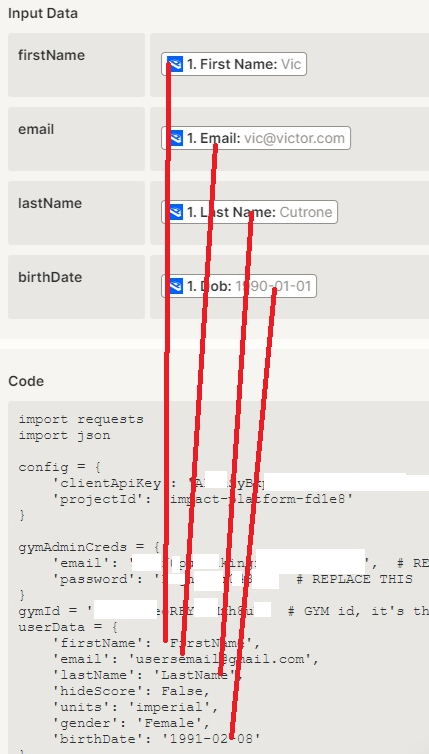
import requests
import json
config = {
'clientApiKey': 'XXX',
'projectId': 'impact-platform-fd1e8'
}
gymAdminCreds = {
'email': 'name@email.com', # REPLACE THIS
'password': 'Password' # REPLACE THIS
}
gymId = '888884444' # GYM id, it's the
userData = {
'firstName': 'FirstName',
'email': 'usersemail@gmail.com',
'lastName': 'LastName',
'hideScore': False,
'units': 'imperial',
'gender': 'Female',
'birthDate': '1991-02-08'
}
def create_user():
try:
signUpResult = requests.post(f"https://identitytoolkit.googleapis.com/v1/accounts:signInWithPassword?key={config['clientApiKey']}",
headers={'Content-Type': 'application/json'},
data=json.dumps({'email': gymAdminCreds['email'], 'password': gymAdminCreds['password'], 'returnSecureToken': True}))
data = signUpResult.json()
if not signUpResult.ok:
error_message = data.get('error', {}).get('message', 'Unknown error occurred')
raise Exception(error_message)
bearer = 'Bearer ' + data['idToken']
createUserResult = requests.post(f"http://us-central1-{config['projectId']}.cloudfunctions.net/iw/functions/gym/{gymId}/user",
headers={'accept': 'application/json', 'authorization': bearer, 'content-type': 'application/json'},
data=json.dumps(userData))
if not createUserResult.ok:
data = createUserResult.json()
error_message = data.get('error', {}).get('message', 'Unknown error occurred')
raise Exception(error_message)
print(f"User {userData['email']} has been created in gym {gymId}")
except Exception as err:
print(err)
create_user()
Best answer by Todd Harper
View original