I’ve been trying to create Zap that can pull metrics like ROAS, AdSpend, Sales, Revenue, and etc. We want to automate that and generate report daily, dumping that to a sheet.
Need someone to correct me because I am not sure if I’m filling it out properly. I’m a beginner so I don’t really have in-depth understanding. Am I using the right trigger and action? TIA
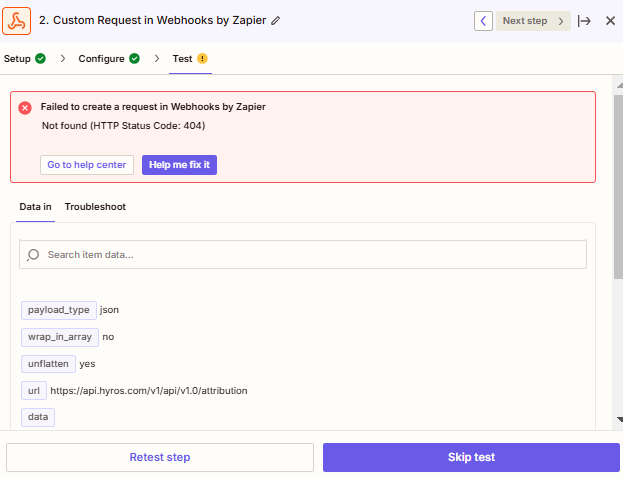
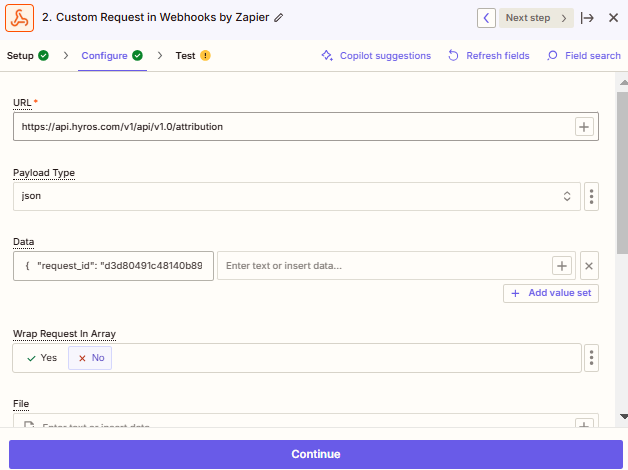
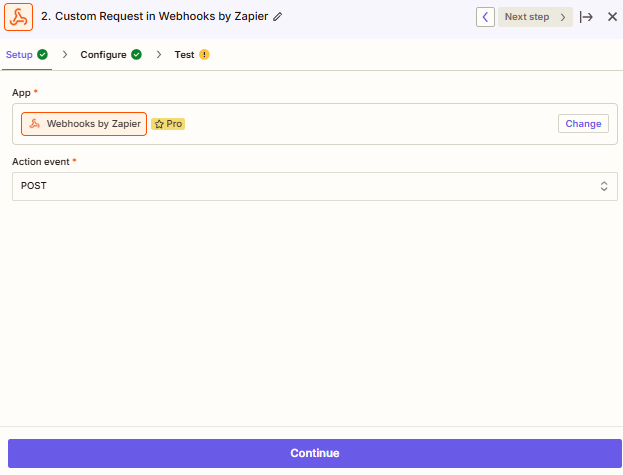
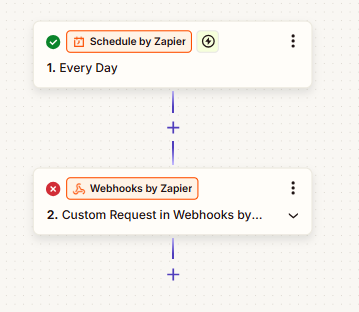