Interesting issue I am encountering here:
I have an action that sends an API request to ClickUp like so:
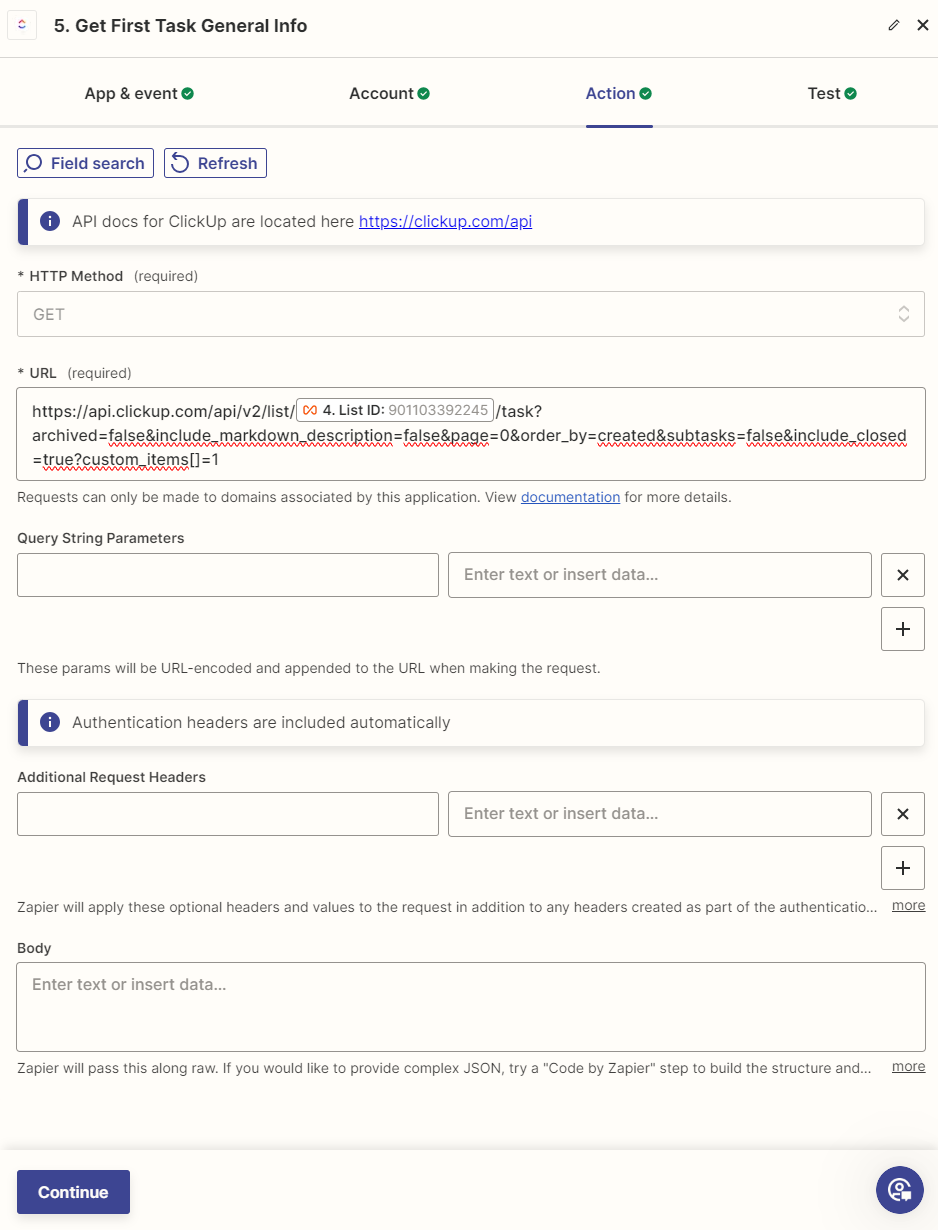
I am interested in parsing out the “TE” and “TT” custom fields values, and the data I get back from the API response appears as such:


As you can see, the data should match up like so:
Field Name | Field Value |
---|---|
QA Issue Status | |
TE | 520 |
TT | 54.549472222222222 |
However, when I go to parse out the index of “TE” and lookup the corresponding value via javascript code, I am noticing that input for value is missing the first comma:
This is my javascript code:
const names = inputData.names.split(',');
const values = inputData.values;
console.log("Names: " + names );
console.log("Values: " + values);
const teIndex = names.findIndex(name => name.includes('TE'));
console.log("TE Index: "+ teIndex);
if (teIndex !== -1){
var workingValues = values;
var indexCount = -1;
var timeEstimatedValue = -1;
while (workingValues.indexOf(',') !== -1){
indexCount++;
const commaIndex = workingValues.indexOf(',');
const temp = workingValues.substring(0, commaIndex);
console.log("Comma Index: " + commaIndex)
console.log("Temp Value: " + temp)
if (teIndex === indexCount){
console.log("FOUND!")
output = { value: temp }
break;
}
workingValues = workingValues.slice(commaIndex + 1)
console.log("Remaining Values: " + workingValues);
}
}
output = { value: "-1" }
This is the output of the tested step:
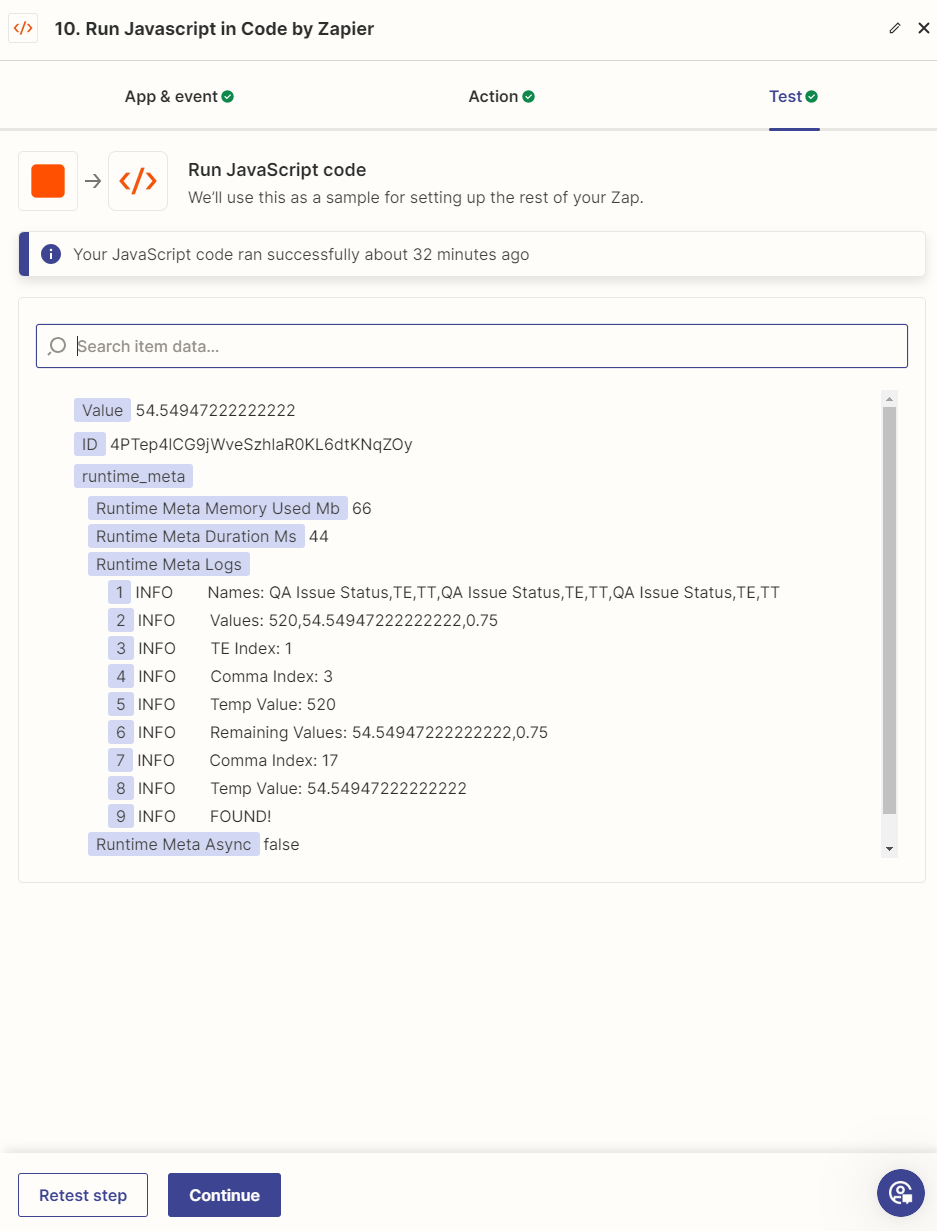
As you can see, Console logs the “values” input to be missing that initial comma that when looking at the response data is there.
What is possibly causing that comma to be stripped?