How to Delay for 1-30 Seconds using JavaScript/Python Code Step
* Use at your own discretion!
Contribution by Troy Tessalone
Premier Certified Zapier Expert at Automation Ace.
OVERVIEW
Zapier implemented these constraints for the Delay Zap app.
- Minimal Delay: From January 13, 2025, the shortest delay you can set for a Zap is 1 minute.
- Automatic Adjustments: If you have any existing Zaps with delays shorter than 1 minute, they will automatically adjusted to 1 minute to prevent any performance impact.
LIMITS
Why 30 seconds MAX?
Zapier has rate limits of 30 seconds MAX for these plans: Professional, Team
Info: https://help.zapier.com/hc/en-us/articles/29971850476173-Code-by-Zapier-rate-limits

PYTHON
Python Code for how to delay between 1-30 seconds.
CODE
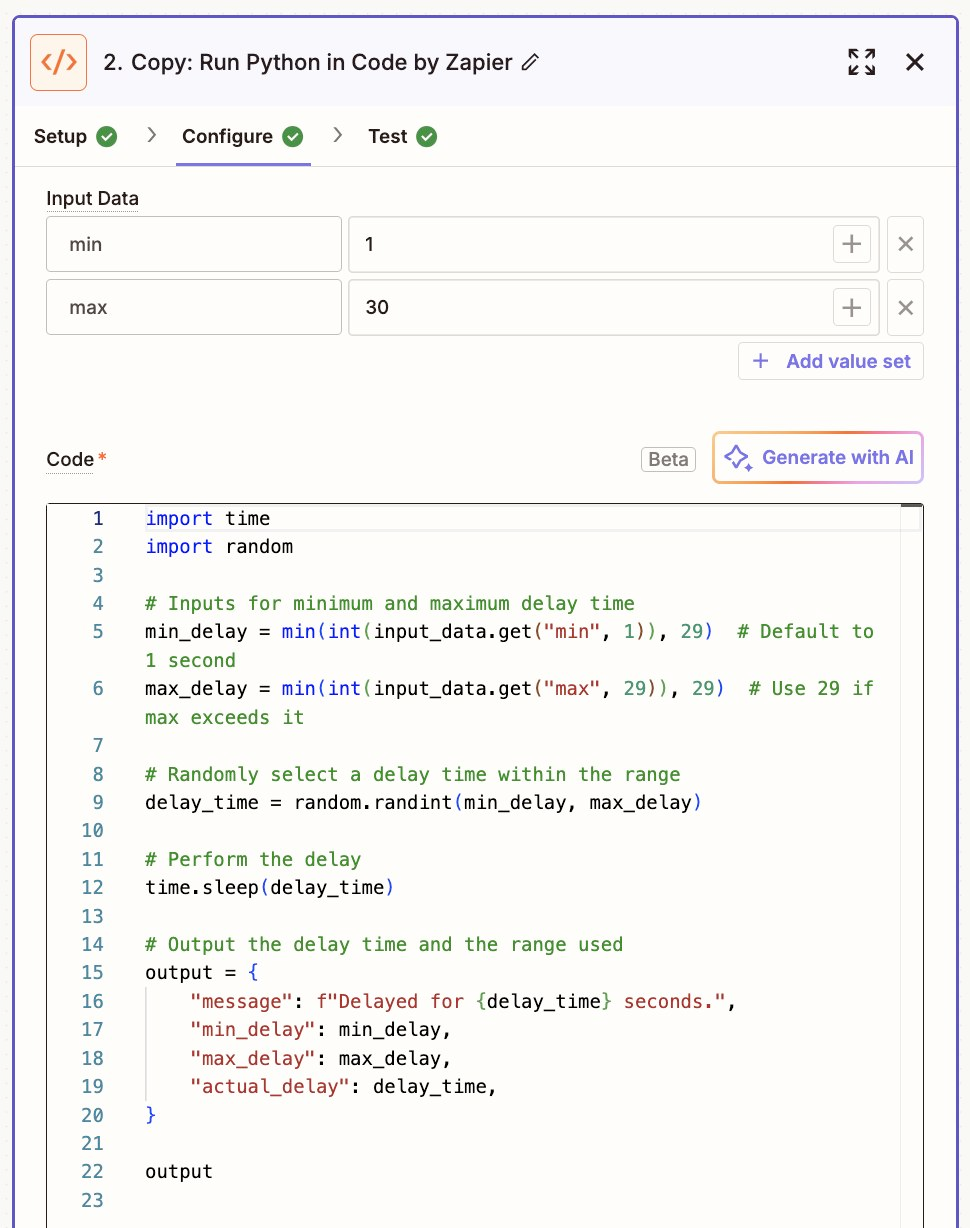
import time
import random
# Inputs for minimum and maximum delay time
min_delay = min(int(input_data.get("min", 1)), 29) # Default to 1 second
max_delay = min(int(input_data.get("max", 29)), 29) # Use 29 if max exceeds it
# Randomly select a delay time within the range
delay_time = random.randint(min_delay, max_delay)
# Perform the delay
time.sleep(delay_time)
# Output the delay time and the range used
output = {
"message": f"Delayed for {delay_time} seconds.",
"min_delay": min_delay,
"max_delay": max_delay,
"actual_delay": delay_time,
}
output
JAVASCRIPT
JavaScript Code for how to delay between 1-30 seconds.
CODE
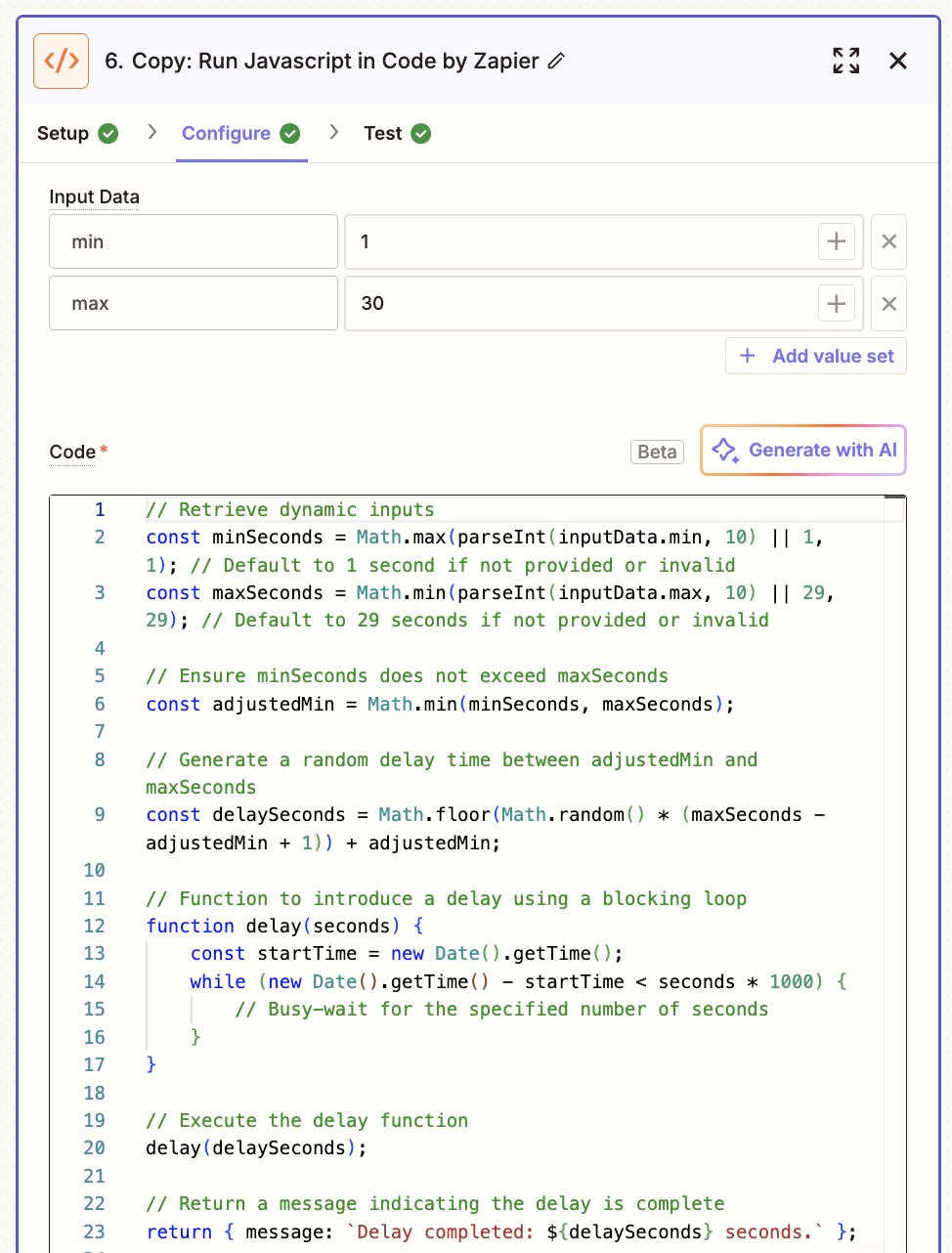
// Retrieve dynamic inputs
const minSeconds = Math.max(parseInt(inputData.min, 10) || 1, 1); // Default to 1 second if not provided or invalid
const maxSeconds = Math.min(parseInt(inputData.max, 10) || 29, 29); // Default to 29 seconds if not provided or invalid
// Ensure minSeconds does not exceed maxSeconds
const adjustedMin = Math.min(minSeconds, maxSeconds);
// Generate a random delay time between adjustedMin and maxSeconds
const delaySeconds = Math.floor(Math.random() * (maxSeconds - adjustedMin + 1)) + adjustedMin;
// Function to introduce a delay using a blocking loop
function delay(seconds) {
const startTime = new Date().getTime();
while (new Date().getTime() - startTime < seconds * 1000) {
// Busy-wait for the specified number of seconds
}
}
// Execute the delay function
delay(delaySeconds);
// Return a message indicating the delay is complete
return { message: `Delay completed: ${delaySeconds} seconds.` };