Tim here from the Zapier Support Team with a workflow idea!
Background
If you’re familiar with the Code by Zapier app, you’re probably used to working with all kinds of values, including arrays. In Zapier, arrays are generally called “Line Items” and sometimes it can be tricky to get them into a Code Step unscathed to run them through some custom process.
Why is it difficult? All values added to the `Input Data` fields of a Code Step are converted into Strings and sent into the Code Step in that format. Arrays / Line Items get converted into Strings that are comma separated values. While it’s definitely possible to split those comma separated values back into arrays inside the code, we run into problems if the values themselves contain commas.
To further complicate things, if we’re sending multiple properties of line items (multiple arrays that relate to each other) and some of the arrays have `null` or empty values, those will be completely dropped, so it can cause our input data to become misaligned.
So how can we work around this?
The Feature Request
First, please write in to support to ask to add your voice to the feature request that we have open to support proper line item input in the Input Data
fields for the Code by Zapier Actions.
The Solution
For now, we can use the Formatter by Zapier app’s Utilities Action: Line-item to Text Transform to help us out.
Note: The Code below is in Javascript but this can be adapted for Python Code Steps as well.
Let’s look at our input values first:
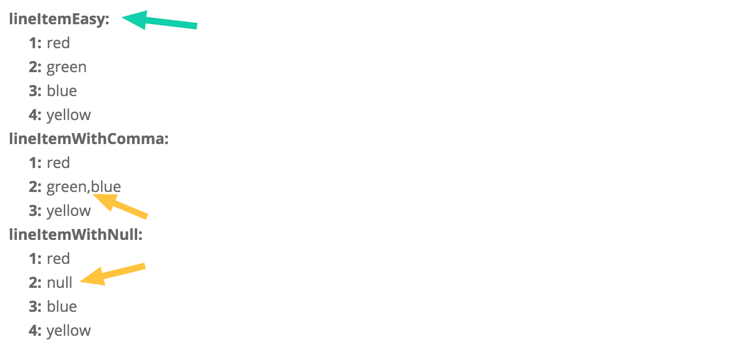
I’ve added an “easy” input first to show how we’d normally get arrays into a Code Step where we don’t need more help. There is also an example with a comma in an element, and another with a null element.
Here’s our default Code Step trying to turn the strings back into arrays:
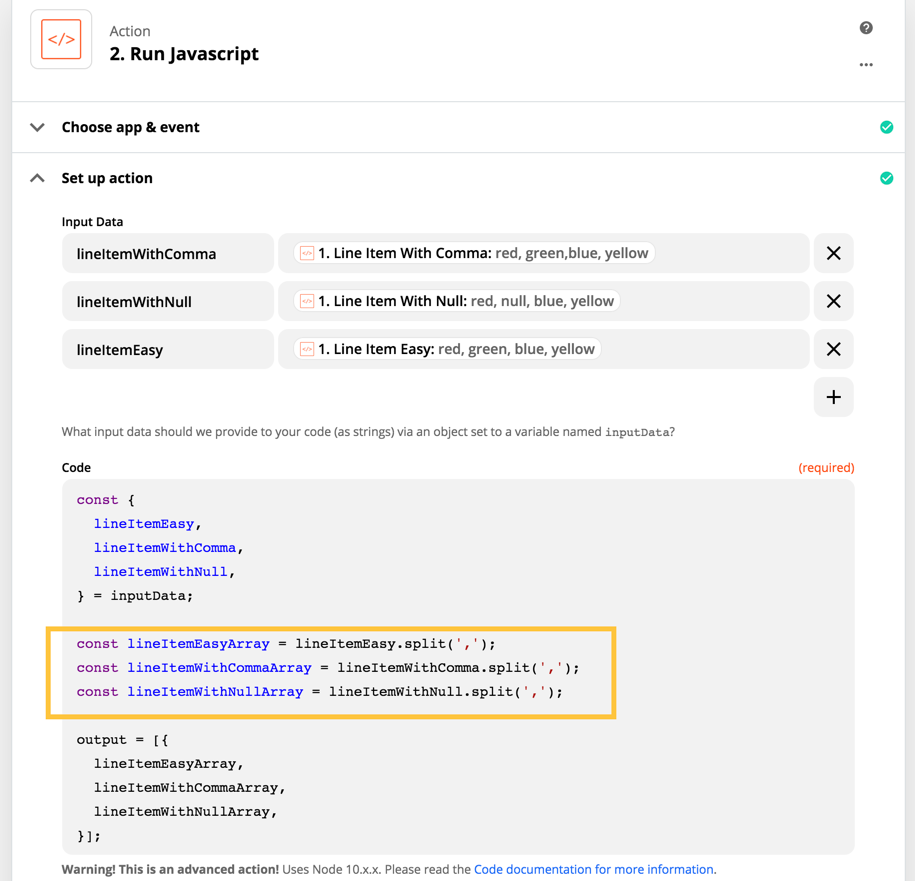
Let’s take a look at the output:
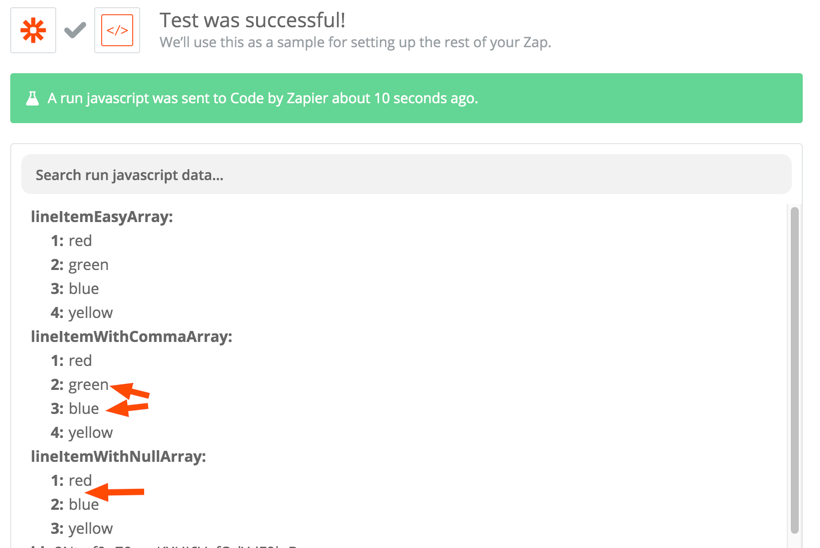
As we can see, the array with comma containing element now has 4 elements instead of 3 as the green,blue
element was split up. The array with the null element has lost that element, reducing it from 4 elements to 3.
Let’s add a Formatter Utilities Action: Line-item to Text Transform to add our own delimiter:
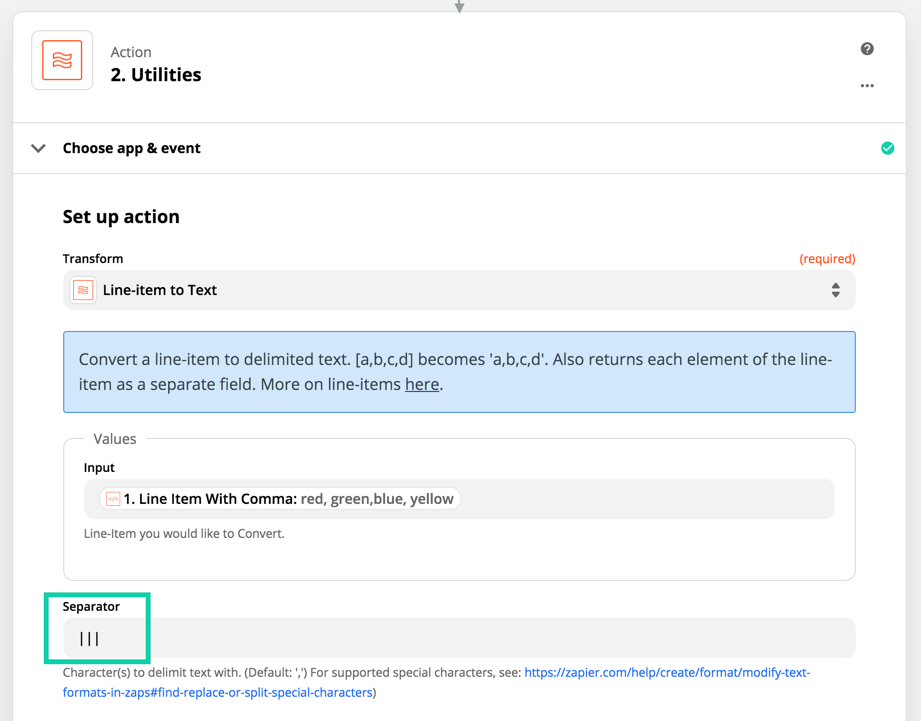
This gives us a text output with a different delimiter:
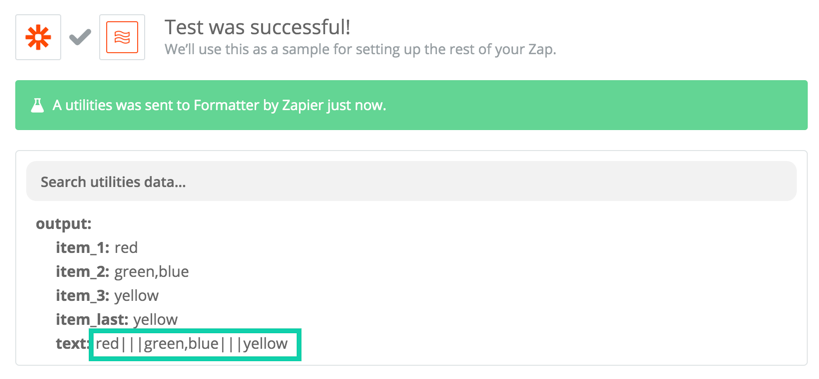
Now we can run this through the Code Step:
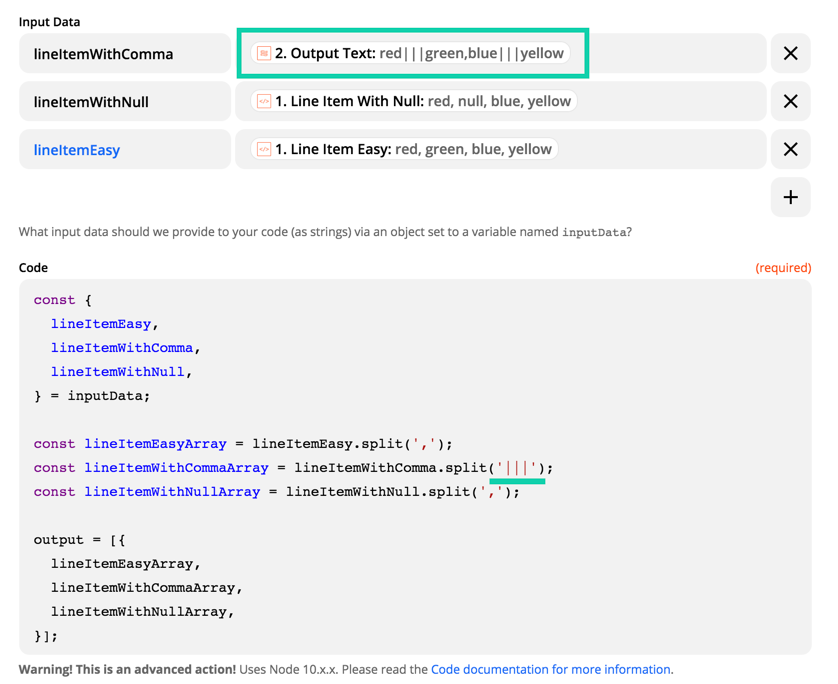
And we get the result we wanted where the value with a comma doesn’t get split into multiple elements:
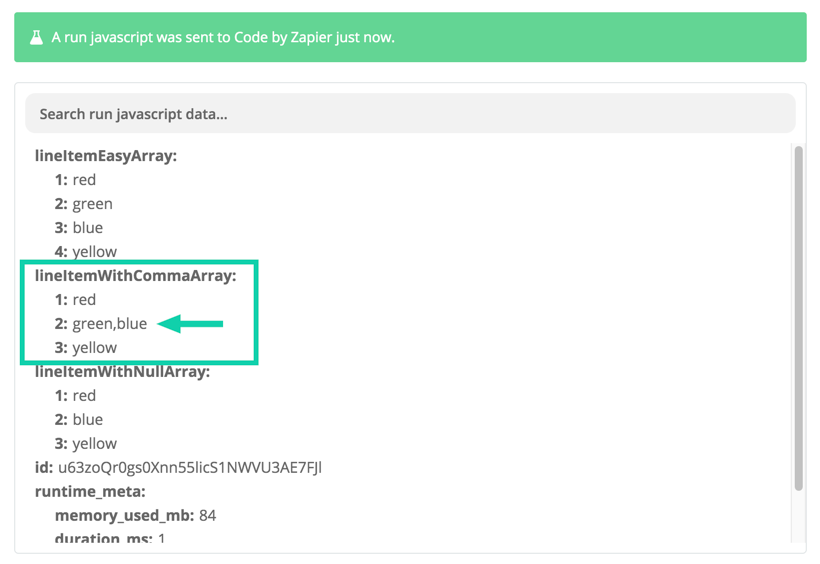
Let’s go back and create another Formatter Utilities Action: Line-item to Text Transform to take care of the array with the null value in it. This time, we also add a `-` after the line item input.
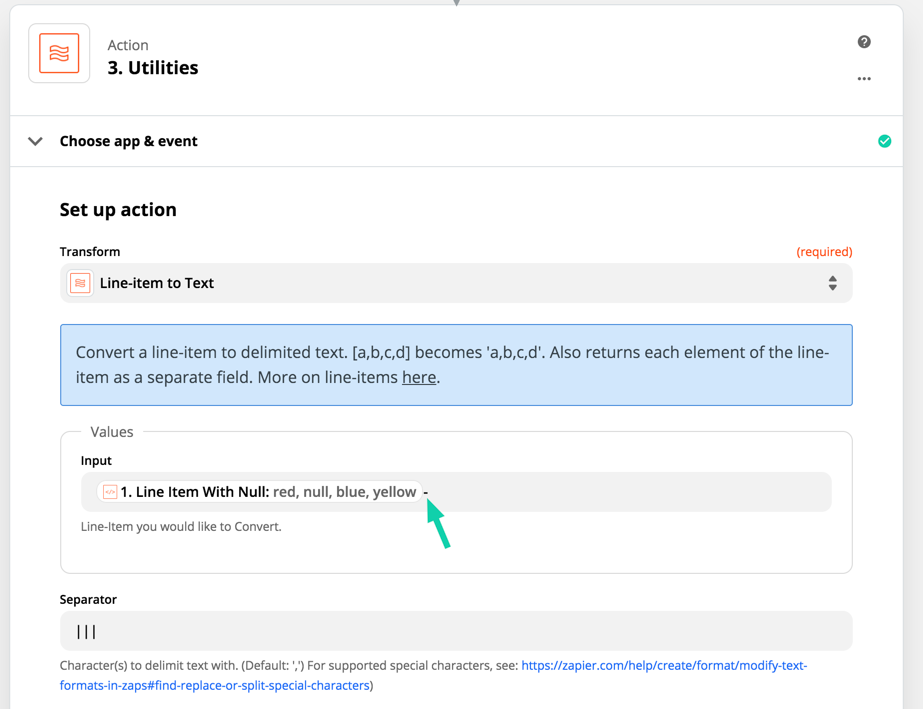
It could be any single character, and what it does is it adds the text -
to each element of the line item/array, even the null elements, so that those aren’t dropped. Here’s the output:
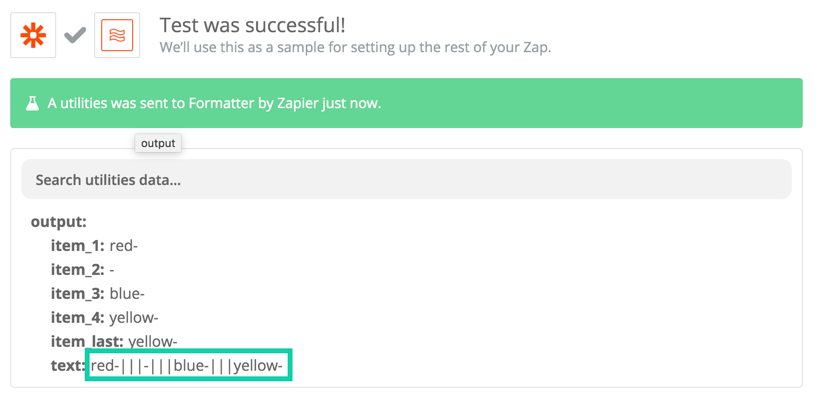
Now we can split on our custom delimiter and then remove the last character from each element inside the Code Step. Here’s how we do that:
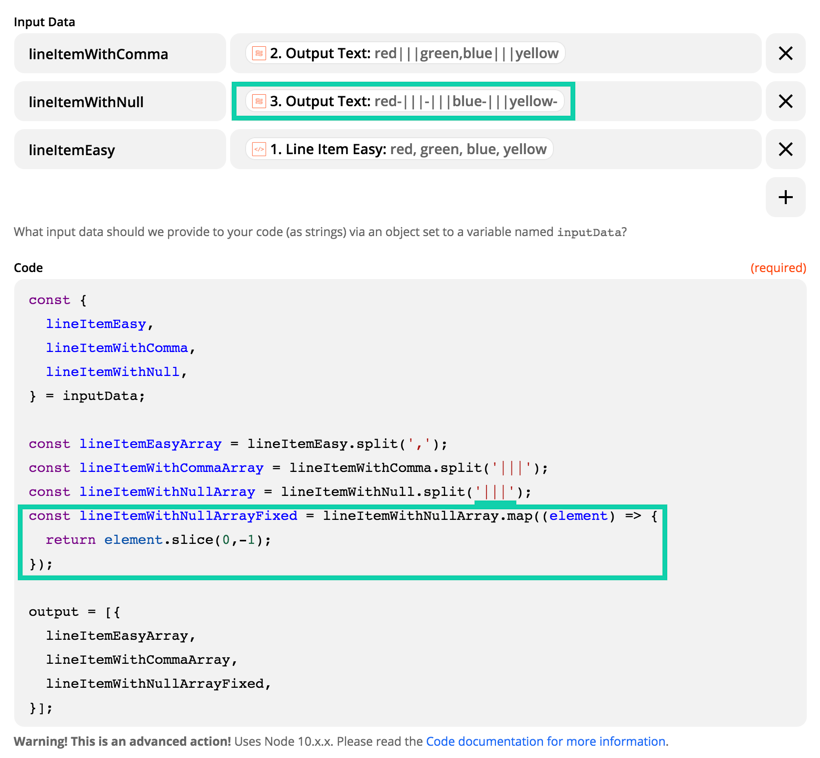
The slice(0,-1) returns the string with the last character removed, so we can get back to our original elements. Here’s our fixed output with the null element in tact:
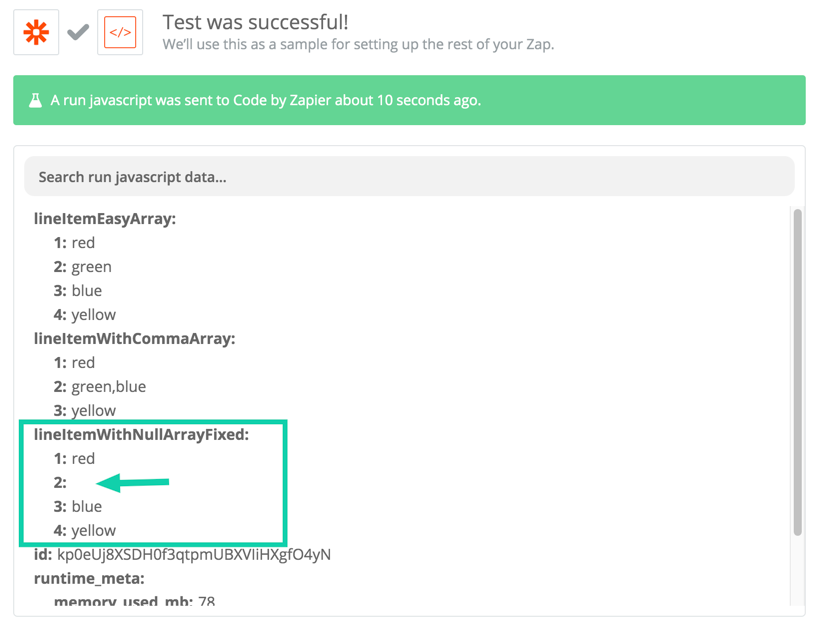
Here’s that final code snippet if you want to copy it and try it out:
const {
lineItemEasy,
lineItemWithComma,
lineItemWithNull,
} = inputData;
const lineItemEasyArray = lineItemEasy.split(',');
const lineItemWithCommaArray = lineItemWithComma.split('|||');
const lineItemWithNullArray = lineItemWithNull.split('|||');
const lineItemWithNullArrayFixed = lineItemWithNullArray.map((element) => {
return element.slice(0,-1);
});
output = t{
lineItemEasyArray,
lineItemWithCommaArray,
lineItemWithNullArrayFixed,
}];
Once you have your Line item values inside your code step with all elements preserved properly, it will be easier to write code to process them in various ways.
Bonus: For a slightly more advanced approach to the above problem but for multiple, related line-item properties, you can also take a look at the answer to the question that inspired this post here:
I hope these workarounds help you make more use of the Code by Zapier app!
Tim
Please Note
This code is provided as is, without warranty, but we’ll do our best to answer questions you have about it here.
Supporting custom code is outside of the scope of Zapier Support, so if you need help with this, or other code you’re working on, the best place to ask is here in the Zapier community and/or on Stack Overflow. You’re still welcome to ask in Support via our contact form just in case it’s something unrelated to the code that isn’t working, but you may be directed to one of these resources to get help from the community.